Last updated : 20- July 2020 01:21 AM
ZOHO has updated all API and now we are not able to run or execute old API so we must need to redirect into Version 2. So I already done ZOHO V2 contact API upgrade task and know how to can setup and update existing or new ZOHO V2 API.
Below is example of ZOHO V2 adding new contact or update existing contact using ZOHO id within a 5 simple step and code.
Step 1:
On first step you need to create a ZOHO Application. If you not created then please create by using below link
https://accounts.zoho.com/developerconsole
Now you click on add Client ID and see below screen
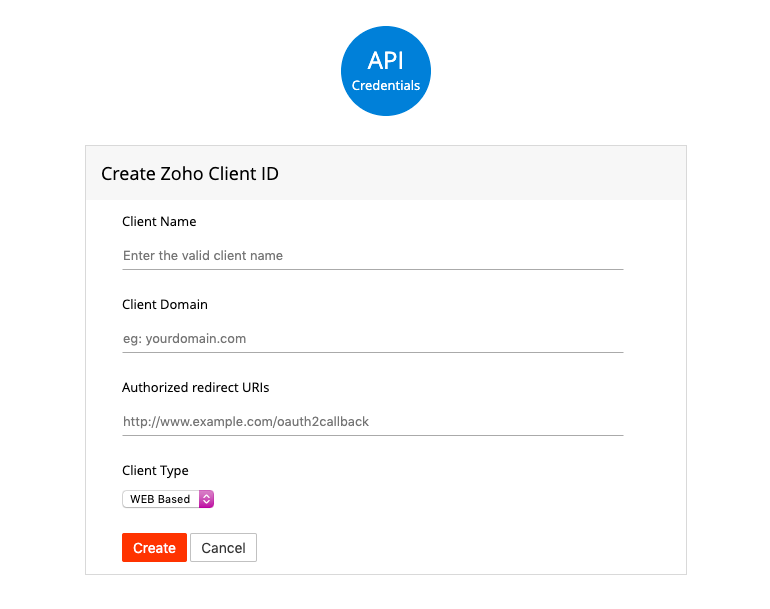
Create a authorisation URL and set correct same redirect URL as Application and on below link. zoho/auth-link.php
https://accounts.zoho.com/oauth/v2/auth?scope=ZohoCRM.modules.all&client_id={CLIENT_ID}&response_type=code&access_type=offline&redirect_uri=https://9code.info/zoho_redirect.php
<a href="{ABOVE LINK}"> Authorise First Time </a>
Step 2:
Now we writing code on zoho/zoho_redirect.php file for generate onetime Grant token based on above link Code response
<?php
error_reporting(E_ALL); ini_set('display_errors', 1);
require 'vendor/autoload.php';
//ZOHO V2 custom class for manage function
require 'zoho_execute.php';
if(!empty($_REQUEST['code'])){
$zoho=new ZOHO_Exec();
$zoho->zoho->generatToken($_REQUEST['code']);
//You can get code using Application as well without creating link and authorise.
}
?>
So once you redirect from ZOHO after executing authorise url you will see on your folder one zcrm_oauthtokens.txt file created. If that is created then that mean you are connected with ZOHO and please do not edit or delete that file otherwise all automation will break.
You can generate code directly using APP as well. need to add Scope and Expiry time:
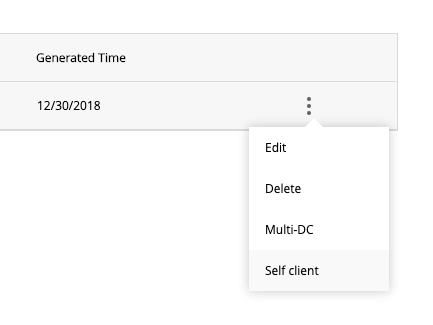
Using above code “zcrm_oauthtokens.txt” name ZOHO token file created and update according to all request using SDK:
Step 3:
On above code we included vendor/autoload.php file that is ZOHO SDK and you can download from https://github.com/zoho/zcrm-php-sdk
You need to execute command on that SDK is composer update so composer will generate vendor folder.
Step 4:
Now Below I describe you zoho/ZohoV2.php class. (Updated as per new SDK version)
<?php
require 'vendor/autoload.php';
use zcrmsdk\oauth\ZohoOAuth;
use zcrmsdk\crm\crud\ZCRMInventoryLineItem;
use zcrmsdk\crm\crud\ZCRMJunctionRecord;
use zcrmsdk\crm\crud\ZCRMNote;
use zcrmsdk\crm\crud\ZCRMRecord;
use zcrmsdk\crm\crud\ZCRMModule;
use zcrmsdk\crm\crud\ZCRMTax;
use zcrmsdk\crm\setup\restclient\ZCRMRestClient;
use zcrmsdk\crm\setup\users\ZCRMUser;
use zcrmsdk\crm\crud\ZCRMCustomView;
use zcrmsdk\crm\crud\ZCRMTag;
use zcrmsdk\crm\exception\ZCRMException;
class ZohoV2 {
private $oAuthClient=null;
private $configuration=null;
public function __construct($conf=null) {
if($conf!=null)
$this->configuration=$conf;
//Initialize Core SDK library
ZCRMRestClient::initialize($this->configuration);
$this->oAuthClient = ZohoOAuth::getClientInstance();
}
//For set all configurations
public function setConfiguration($config){
$this->configuration=$config;
}
// When after authorization you redirect you will get this grantToken and using that you will get access token
public function generateToken($grantToken){
//Below is sample grant token
// $grantToken = "1000.11355e7151087bf58933e82c3876a5e9.609bbf22b49c1d43b36fbe3708b2399c";
return $oAuthTokens = $this->oAuthClient->generateAccessToken($grantToken);
}
//This function if access token expire then using refresh token you can generate new Access token
public function refreshToken($refreshToken,$userIdentifier){
//below is sample refresh token you found and that automatically updated once generate
return $oAuthTokens = $this->oAuthClient->generateAccessTokenFromRefreshToken($refreshToken,$userIdentifier);
}
//Update existing record
public function updateRecord($zohoid,$data,$module="Contacts"){
try{
$zcrmRecordIns = ZCRMRecord::getInstance($module, $zohoid);
foreach($data as $d=>$v){
$zcrmRecordIns->setFieldValue($d, $v);
}
$entityResponse=$zcrmRecordIns->update();
if("success"==$entityResponse->getStatus()){
$createdRecordInstance=$entityResponse->getData();
return $createdRecordInstance->getEntityId();
echo "Status:".$entityResponse->getStatus();
echo "Message:".$entityResponse->getMessage();
echo "Code:".$entityResponse->getCode();
echo "EntityID:".$createdRecordInstance->getEntityId();
echo "moduleAPIName:".$createdRecordInstance->getModuleAPIName();
}
return "";
}catch(Exception $e){
$file_names = __DIR__."/zoho_ERROR_UPDATE_log.txt";
file_put_contents($file_names, $e.PHP_EOL , FILE_APPEND | LOCK_EX);
return "";
}
}
//Create a new contact record
public function newRecord($data,$module="Contacts") {
//if(count($data)<=0)return "";
$records = [];
try{
$record = ZCRMRecord::getInstance( 'Contacts', null );
foreach($data as $d=>$v)
$record->setFieldValue($d, $v);
$records[] = $record;
$zcrmModuleIns = ZCRMModule::getInstance($module);
$bulkAPIResponse=$zcrmModuleIns->createRecords($records); // $recordsArray - array of ZCRMRecord instances filled with required data for creation.
$entityResponses = $bulkAPIResponse->getEntityResponses();
foreach($entityResponses as $entityResponse){
if("success"==$entityResponse->getStatus()){
$createdRecordInstance=$entityResponse->getData();
return $createdRecordInstance->getEntityId();
}
else{
$file_names = __DIR__."/zoho_ERROR_ADDNEW_log.txt";
file_put_contents($file_names, (json_encode($entityResponses)).PHP_EOL , FILE_APPEND | LOCK_EX);
return "-1";
}
}
}catch(Exception $e){
$file_names = __DIR__."/zoho_ERROR_ADDNEW_log.txt";
file_put_contents($file_names, $e.PHP_EOL , FILE_APPEND | LOCK_EX);
return "";
}
}
// GEt specific record by using id
public function getRecordById($id=1000266000007206013,$module="Contacts"){
$zcrmModuleIns = ZCRMModule::getInstance($module)->getRecord($id);
return $recordsArray = $zcrmModuleIns->getData();
}
}
?>
Okay so above class is created for us to manage action with using PHP SDK v2 now API code library has Setup and we can access that using below code.
Step 5:
Let’s execute above library code in zoho/zoho_execute.php file
<?php
include ("ZohoV2.php");
class ZOHO_Exec{
public $zoho;
function __construct(){
$configuration=
array(
"client_id"=>"<CLIENT ID>",
"client_secret"=> "<SECRATE CODE>",
"redirect_uri"=>"<REDIRECT BASE URL>/zoho_redirect.php",
"currentUserEmail"=> "<ACC EMAIL>",
"access_type"=>__DIR__."/",
"persistence_handler_class"=>"ZohoOAuthPersistenceHandler",
"token_persistence_path"=>__DIR__."/",
"applicationLogFilePath"=>__DIR__."/",
"user_email_id"=>"<SUB USER>"
);
$this->connect_zoho($configuration);
}
private function connect_zoho($conf){
$this->zoho = new ZohoV2($conf);
return true;
}
public function zoho_data($row=null){
/* Here you need to map your ZOHO fields with value more customization please contact [email protected] for any PHP framwork or in CMS */
if(empty($row))
$row = array(
'First_Name'=>'parbat',
'Last_Name'=>'pithiya',
'Email'=>'[email protected]',
'Company'=>'Gatetouch',
'Mobile'=>'9998873404',
'Mailing_Street'=>'C-235,siddharth excellence',
'Mailing_City'=>'Vadodara',
'Mailing_Zip'=>'390015',
'Mailing_State'=>'Gujarat',
'Country'=>'India',
'Subscription_Status'=>'Active',
'Lead_Source'=>'9code.info',
'Coupon'=>'ALL_FREE'
);
return $row;
}
private function zohoupdate($row=null,$zohoid=1234567890){
// Get ZOHO data
$lead = $this->zoho_data($row);
if(!empty($zohoid) && intval($zohoid)>0) {
$this->zoho->updateRecord( $zohoid,$lead);
} else {
//new registration
$zohoid = $this->zoho->newRecord($lead);
$this->set_zoho_id($row, $zohoid);
}
}
private function set_zoho_id($row,$zohoid){
/*
Here you can update your record with new zoho ID so next time you can update zoho record
*/
}
}
?>
Step 6:
On Step 6 we will execute our operation like view, insert, update and delete into zoho.
<?php
include("zoho/zoho_execute.php");
//Now calling above class object
$exeZ =new ZOHO_Exec();
//Now going to add record in contact module
var_dump( $exeZ->zoho->newRecord($exeZ->zoho_data()));
Finally we have created new record in contact module you can also set other parameters in this function as a module API Name eg. Contacts, Accounts, …
Thank you for the using my code.
If you having any issue while configuring or you want my help to connect your Application with ZOHO v2 API.
May be you need to edit Core SDK file if that is not working then /vendor/zohocrm/php-sdk/src/oauth/ZohoOAuthClient.php
In SDK i have find some issues on line no 158
return (isset($jsonResponse['Email']))?$jsonResponse['Email']:'';
Thank you and comment here if you still causing any issues. you are the best 🙂
In step 5, there is a line “include __DIR__ . ‘/zohoSDK/zoho.php’;” under function connect_zoho().
I want to know where this file is / or what is the code inside this file?
Hey Birendra
that is file is ZohoV2.php
I have started implementing as instructed and found that the script is not working as aspected througing many errors.
Can you please provide a zip with a sample demo so that we just replace the client id and secret key and it start working?
Hey Momin,
Sure i will help you, can you please share issues what you having while configuring above code. you can skype me as well on parbat_pithiya i will
see your code and help you 🙂
https://help.zoho.com/portal/kb/zoho-crm/developer-guide/server-side-sdks/php-sdk
The requested page could not be found.
:-/
Yes they have removed that page
Now you can see full details on below PDF (v1)
https://www.zoho.com/crm/downloads/php-version1.pdf
Also you will get code
https://github.com/zoho/zcrm-php-sdk
Hello, where i can find the class ZohoOAuthPersistenceHandler, i don´t understand the use Persistence handler.
We need have a ZohoOAuthPersistenceHandler class ?
Do you have a complete code of project?
Thank you
This is complete code and all working just you need to configure properly Auth Code. Once done this will auto metic generate token using Refresh Token. and even not then drop me error message.
ZohoOAuthPersistenceHandler is used for store and manage Zoho Tokens.
Thanks
Buenas.
Me llamo Jorge y al ejecutar el programa lo redirecciono al ZOHO_exce verdad?
Pero al hacerlo no guarda la informaciĂłn
Y en la documentacion no encuentro el user_email_id y el CurrenEMail
Por favor ayuda.
GRacias
Hi Jorge,
Redirect url need to set zoho_redirect.php.
user_email_id is your ZOHO account email and that is store into your persistence token files (zcrm_oauthtokens.txt).
Thanks
Where do I put the zcrm_oauthtokens.txt file? what is its content?
what is the correct configuration of token persistence by file?
In the step 5, the code, where i can put the code step 5?
what is the name of file step 5?
thank you
Step 5 code is a class and i have executed that class object at end of class and updating record. So in url directly you need to execute that file
Hello, can you publish the content of file zcrm_oauthtokens.txt and where you call this file in SDK PHP
On that file CRM will generated dynamic serialise format data
Hey Dear, Thanks for the tutorial in Advance, but it seems that there are many error in this code or in my configuration.
I have copied your code as per given instruction and replace the configuration details too.
Please check the screenshot for folder structure:
https://drive.google.com/file/d/1BXOqR9zSCok0Qqk-3RqOIW6SeWxapZAt/view?usp=sharing
index.php having :
screenshot 1: https://drive.google.com/file/d/1NrOZxDu2Pq3O8BkgqlX4McJkIUcV11K0/view?usp=sharing
zoho_execute.php having:
screenshot 1: https://drive.google.com/file/d/1pg_dnOUxI3V9K2tgy6Lb2VRjnZdbZXWi/view?usp=sharing
screenshot 2: https://drive.google.com/file/d/1RvMG8LZrjMNDGCTvq0TqHdSTFbK8AkXa/view?usp=sharing
screenshot 3: https://drive.google.com/file/d/15e0KV2ihyyezKcAKpFGi0MZkxa9g9y3p/view?usp=sharing
screenshot 4: https://drive.google.com/file/d/1zowQy9YVVs1TPZZ-zXaZE6Dwn1nAyoCk/view?usp=sharing
zoho_redirect.php having:
screenshot 1: https://drive.google.com/file/d/1_lrUP5jo5P3ytofRsqwFJH6oFteKeOaf/view?usp=sharing
ZohoV2.php having:
screenshot 1: https://drive.google.com/file/d/1obq8yDfUpxfJjRE1872ufSFwo1gkNSNe/view?usp=sharing
screenshot 2: https://drive.google.com/file/d/1YSktPz5gMxSTMwt8XUQARgjZUWZi_mU0/view?usp=sharing
screenshot 3: https://drive.google.com/file/d/13sTE1faQ1Yfa4aADCmw_pfxLXRIQrz9n/view?usp=sharing
screenshot 4: https://drive.google.com/file/d/1RPRAeNss76zby55hua7twjZmA-q0M4c-/view?usp=sharing
Every details i.e. Client ID and Client Secret seems to be fine.
I have click on “Authorise First Time” and it is successfully redirecting to zoho_redirect.php
Then i am executing zoho_execute.php file to make zoho api in action, but no luck
Everything seems to be fine as per tutorial, but not working. can you please investigate and check what am i missing to make it working?
How i proceed with this code?
Today I have updated code as per latest SDK version.
So please recheck above working code and let me know if having any issue.
Hello sir, Can you help me Solve my error.
zcrmsdk\oauth\exception\ZohoOAuthException Caused by:’No Tokens exist for the given user-identifier,Please generate and try again.’ in /home/netsh9ro/public_html/grivance/API/vendor/zohocrm/php-sdk/src/oauth/persistence/ZohoOAuthPersistenceByFile.php(46)
#0 /home/netsh9ro/public_html/grivance/API/vendor/zohocrm/php-sdk/src/oauth/ZohoOAuthClient.php(37): zcrmsdk\oauth\persistence\ZohoOAuthPersistenceByFile->getOAuthTokens(‘rrjjaiswal7052@…’)
#1 /home/netsh9ro/public_html/grivance/API/vendor/zohocrm/php-sdk/src/crm/utility/ZCRMConfigUtil.php(98): zcrmsdk\oauth\ZohoOAuthClient->getAccessToken(‘rrjjaiswal7052@…’)
#2 /home/netsh9ro/public_html/grivance/API/vendor/zohocrm/php-sdk/src/crm/api/APIRequest.php(71): zcrmsdk\crm\utility\ZCRMConfigUtil::getAccessToken()
#3 /home/netsh9ro/public_html/grivance/API/vendor/zohocrm/php-sdk/src/crm/api/APIRequest.php(113): zcrmsdk\crm\api\APIRequest->authenticateRequest()
#4 /home/netsh9ro/public_html/grivance/API/vendor/zohocrm/php-sdk/src/crm/api/handler/MassEntityAPIHandler.php(56): zcrmsdk\crm\api\APIRequest->getBulkAPIResponse()
#5 /home/netsh9ro/public_html/grivance/API/vendor/zohocrm/php-sdk/src/crm/crud/ZCRMModule.php(1177): zcrmsdk\crm\api\handler\MassEntityAPIHandler->createRecords(Array, NULL, NULL)
#6 /home/netsh9ro/public_html/grivance/API/zoho/ZohoV2.php(103): zcrmsdk\crm\crud\ZCRMModule->createRecords(Array)
#7 /home/netsh9ro/public_html/grivance/API/zoho/index.php(6): ZohoV2->newRecord(Array)
#8 {main}
Can you please go into /vendor/zohocrm/php-sdk/src/oauth/ZohoOAuthClient.php
In SDK i have find some issues on line no 158
return (isset($jsonResponse[‘Email’]))?$jsonResponse[‘Email’]:”;
Hi,
I’m using your code. It works well.
But, right bnow i’m facing problem.
can you help me to display all Leads with only fields and values along with criteria like Lead_source=’xxx’ and if possible with pagination.
Thank You
Sure we will help you on this.
Hi,
i use your code and it’s work well but first time when i get grant token it will insert into token file but after one hour it is expire and record is not insert into zoho it will return an error like “Access Token has expired. Hence refreshing.”. can you help me?
Mail me on [email protected] with details, will help you
Hello, i think you did not get refresh token, so generate grant token with the grant type ZohoCRM.modules.all,ZohoCRM.settings.ALL and use that token by execution of redirect.php?code= so using this way you will get all token and never expire token.
Also need to set Correct timezone in your server so get right expiration time
hello, i’ve sent you an email with question on your paypal email about the purchase, do you have another email?
Mail me on [email protected] with details, will help you
As you said at the end of the article, I changed line 158 on “ZohoOAuthClient.php” but it still shows this error:
“No Tokens exist for the given user-identifier, Please generate and try again.”
Currently, the file “zcrm_oauthtokens.txt” is created and filled.
I tried to debug it and from my test page which the “zoho_execute.php” is included, I start to find the issue:
1. This function works well: $exeZ->zoho_data
2. Also this function is being executed: $exeZ->zoho->newRecord
3. Then “$this->connect_zoho” is being called inside the class “__constructor” of “ZOHO_Exec”
4. Inside the function “connect_zoho”, this class “ZohoV2” is being created
5. Inside the class “ZohoV2” the “__construct” is being called and after initializing the configuration, “ZohoOAuth::getClientInstance” is being executed and put the result into the “oAuthClient”
I don’t know the token is not going to be generated or the issue is with something else.
Hello sir, Can you help me Solve my error,
Fatal error: Uncaught zcrmsdk\oauth\exception\ZohoOAuthException Caused by:’zcrmsdk\oauth\exception\ZohoOAuthException Caused by:’Exception while fetching access token from grant token – HTTP/1.1 200 Server: ZGS Date: Fri, 07 Feb 2020 06:25:21 GMT Content-Type: application/json;charset=UTF-8 Content-Length: 33 Connection: keep-alive Set-Cookie: b266a5bf57=a711b6da0e6cbadb5e254290f114a026; Path=/ X-Content-Type-Options: nosniff X-XSS-Protection: 1 Set-Cookie: iamcsr=c6be67b3-55ae-450a-ae8d-4215199fce14;path=/;Secure;priority=high X-Frame-Options: SAMEORIGIN Strict-Transport-Security: max-age=63072000 {“error”:”invalid_client_secret”}’ in C:\xampp\htdocs\zoho\vendor\zohocrm\php-sdk\src\oauth\ZohoOAuthClient.php(69) #0 C:\xampp\htdocs\zoho\ZohoV2.php(41): zcrmsdk\oauth\ZohoOAuthClient->generateAccessToken(‘1000.0b1a458df9…’) #1 C:\xampp\htdocs\zoho\zoho_redirect.php(12): ZohoV2->generateToken(‘1000.0b1a458df9…’) #2 {main}’ in C:\xampp\htdocs\zoho\vendor\zohocrm\php-sdk\src\oauth\ZohoOAuthClient.php(72) #0 in C:\xampp\htdocs\zoho\vendor\zohocrm\php-sdk\src\oauth\ZohoOAuthClient.php on line 72
Hello Parbat,
I downloaded your code and I tried to implement your files, but I don’t understand how to continue.
I launch the index.php file in the browser and I click on the link “Authorize Your Account”.
After then, I click on the green buttons of the Zoho Interface and I return on the screen of the index file.
And then, what am I gonna do, to insert a new Lead on the CRM?
Thank you
Best Regards
Hello Luca,
As per our mail conversation, your issue is solved, thank you
Hi Prabhat,
I have got an error “Exception while fetching access token from refresh token” while i have created run first step file
Yes sometime having issue so in this case we need to generate access token from app panel and pass same as how redirect and passing token after this step you will generate all token file.
Hi prabhat,
Got the error. please help
Fatal error: Uncaught zcrmsdk\oauth\exception\ZohoOAuthException Caused by:’zcrmsdk\oauth\exception\ZohoOAuthException Caused by:’Exception while fetching access token from grant token – HTTP/1.1 200 Server: ZGS Date: Tue, 30 Jun 2020 12:00:15 GMT Content-Type: application/json;charset=UTF-8 Content-Length: 26 Connection: keep-alive Set-Cookie: b266a5bf57=57c7a14afabcac9a0b9dfc64b3542b70; Path=/ X-Content-Type-Options: nosniff X-XSS-Protection: 1 Set-Cookie: iamcsr=bd81e547-567f-4511-93d2-66eef3a8f07b;path=/;SameSite=None;Secure;priority=high Set-Cookie: _zcsr_tmp=bd81e547-567f-4511-93d2-66eef3a8f07b;path=/;SameSite=Strict;Secure;priority=high X-Frame-Options: SAMEORIGIN Strict-Transport-Security: max-age=63072000 {“error”:”invalid_client”}’ in /home/tech11dheeraj/public_html/zohocrm/src/oauth/ZohoOAuthClient.php(71) #0 /zohocrm/ZohoV2.php(41): zcrmsdk\oauth\ZohoOAuthClient->generateAccessToken(‘1000.84a5b8917f…’) #1 /home/tech11dheeraj/public_html/zohocrm/getNewToken.php(2 in zohocrm/src/oauth/ZohoOAuthClient.php on line 74
Still having issue send me mail [email protected]
Hello, I have integrated the sdk with laravel, but I cannot create zcrm_oauthtoken.txt, file_get_contents() error
Please help
That is ZOHO SDK error because of PHP version, you need to user file_get_contents(path of that token file) in SDK and then it will work.