Hello Guys, Looking Standard Code with using Object Oriented Programing Language(OOP) ? OKAY let me describe you demo of login and registration with email verification code. Also i have used latest bootstrap 4.
All project this is first stage to create a login and registration forms with email verification. Now a days you got many examples and demos, with outdated code and functions. I’m trying to describe you step by step.
First Steps is you need to design database:
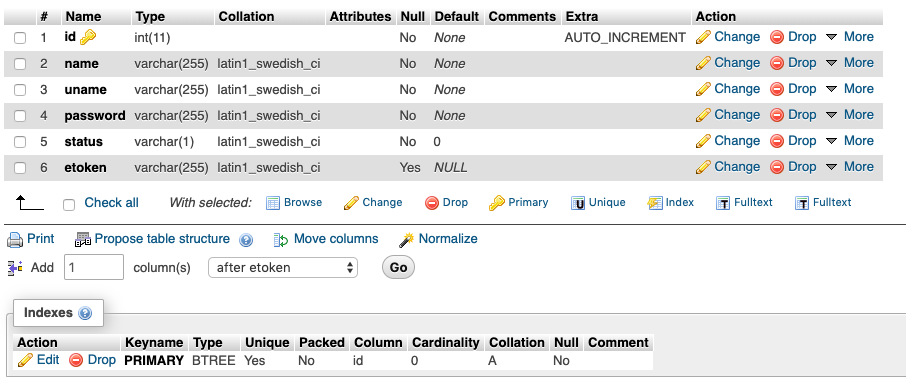
Now Let me export sql script for you to import in your database.
CREATE TABLE `user_master` (
`id` int(11) NOT NULL,
`name` varchar(255) NOT NULL,
`uname` varchar(255) NOT NULL,
`password` varchar(255) NOT NULL,
`status` varchar(1) NOT NULL DEFAULT '0',
`etoken` varchar(255) DEFAULT NULL
) ENGINE=MyISAM DEFAULT CHARSET=latin1;
--
-- Indexes for dumped tables
--
--
-- Indexes for table `user_master`
--
ALTER TABLE `user_master`
ADD PRIMARY KEY (`id`);
--
-- AUTO_INCREMENT for dumped tables
--
--
-- AUTO_INCREMENT for table `user_master`
--
ALTER TABLE `user_master`
MODIFY `id` int(11) NOT NULL AUTO_INCREMENT;
COMMIT;
After executing above query then you need to design structure like this we used Bootstrap 4
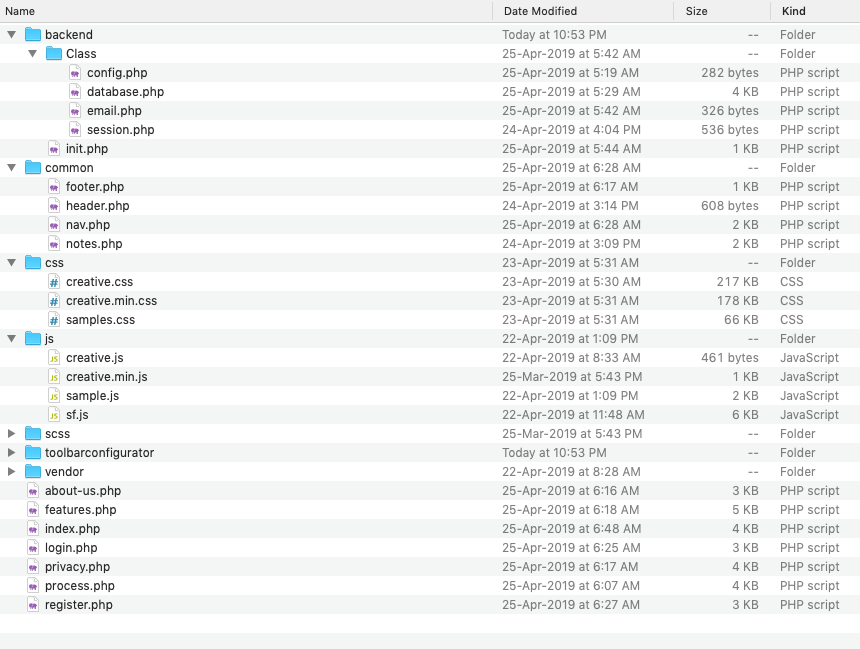
First you need to setup .htaccess file for creating SEO friendly login and registration page access
Options -MultiViews
RewriteEngine On
RewriteCond %{REQUEST_FILENAME} !-f
RewriteRule ^([^\.]+)$ $1.php [NC,L]
So using above htaccess you access access login and registration page eg. /login and /register no need .php extension
Now we creating backend/init.php file as below
<?php
//Including support classes for separate each functions
include(__DIR__."/Class/session.php");
include(__DIR__."/Class/database.php");
include(__DIR__."/Class/email.php");
class app{
public $session=null;
public $db=null;
function __construct(){
//Initializing object of database and session class
$this->initDB();
$this->initSession();
}
private function initDB(){
if(is_null($this->session))
$this->session=new session();
$this->db=new database($this->session);
}
private function initSession(){
$this->session=new session();
}
public function getEmail(){
return new email();
}
public function redirect($url=""){
header("Location:".$this->db->base_url.$url);
}
public function getInput($key,$default=null){
if(isset($_REQUEST[$key])){
return trim($_REQUEST[$key]);
}
return $default;
}
function getMessages(){
$msg=$this->session->get("message");
$msg2=[];
if(isset($msg['type']))
$msg2[]=$msg;
else $msg2=$msg;
if($msg2)
foreach($msg2 as $m){
echo '<div class="alert alert-'.$m['type'].'" role="alert">'.$m['message']."</div>";
}
}
}
/*
Now run the app to accessible object within a project with single connection of database and instance.
*/
$app= new app();
Above class for center communication of all classes and methods. also there we defined common methods for our project reused.
Now we need to create a included all class files and we need to create below three classes
include(DIR."/Class/session.php");
<?php
@session_start();
class session{
static function get($key){
if( isset($_SESSION[$key]))
return $_SESSION[$key];
return null;
}
static function set($key,$value){
if( isset($_SESSION[$key]))
unset($_SESSION[$key]);
$_SESSION[$key]=$value;
}
static function isLogin(){
if(isset($_SESSION['user']) && $_SESSION['user']!=null)
return true;
return false;
}
static function destroy(){
session_destroy();
}
}
include(DIR."/Class/database.php");
<?php
//added database and other static constant config class
include ("config.php");
class database extends config{
public $conn=null;
public $result=null;
public $session=null;
public $insert_id=null;
public $last_insert_id=null;
function __construct($session=null){
$this->connect();
$this->session=$session;
}
function connect(){
$this->conn = new mysqli($this->host, $this->user, $this->password, $this->db);
/* check connection */
if ($this->conn->connect_errno) {
throw new Exception($this->conn->connect_error);
}
}
private function tableExists($table) {
$tablesInDb = $this->conn->query('SHOW TABLES FROM `' . $this->db . '` LIKE "' . $table . '"');
if ($tablesInDb) {
if ($tablesInDb->num_rows == 1) {
return true;
} else {
return false;
}
}
}
/* function result(){
return $this->result->fetch_assoc();
}*/
function select($table,$where_=null,$tsart=null,$litmit=null){
if(!$this->tableExists($table))
return false;
$query="SELECT * FROM $table ".(($where_!=null)?' WHERE ' .$where_:'');
if($tsart!=null && intval($tsart) && $litmit!=null && intval($litmit))
$query.=" LIMIT $tsart,$litmit";
if ($this->result = $this->conn->query($this->last_query=$query)) {
return $this->result;
}
var_dump("asda");
EXIT;
}
function insert($table,$insData){
if(!$this->tableExists($table))return false;
try {
$columns = implode(", ",array_keys($insData));
$escaped_values = array_map(array($this,'escapeValue'), array_values($insData));
$values = implode(", ", $escaped_values);
$sql = "INSERT INTO `$table`($columns) VALUES ($values)";
if ($this->conn->query($this->last_query=$sql) === TRUE) {
return $this->last_insert_id=$this->conn->insert_id;
}
return false;
} catch(Exception $e) {
//$mysqli->rollback(); //remove all queries from queue if error (undo)
throw $e;
}
}
function escape($data){
return "`".$this->conn->real_escape_string($data)."`";
}
function escapeValue($data){
if(is_numeric($data))
return $this->conn->real_escape_string($data);
else
return "'".$this->conn->real_escape_string($data)."'";
}
function update($table,$data,$where_){
try {
if(!$this->tableExists($table))return false;
$update = 'UPDATE ' . $table . ' SET ';
$up=[];
foreach($data as $k=>$v){
if ( $v==NULL) {
$up[] =$this->escape($k) . '=' . $this->escapeValue($v);
}
}
$update .= implode(",",$up);
$update .= (($where_!=null)?' WHERE ' .$where_:'');
$query = $this->conn->query($this->last_query=$update);
if ($query)
return true;
else
return false;
} catch(Exception $e) {
//$mysqli->rollback(); //remove all queries from queue if error (undo)
throw $e;
}
}
function getUser($id=null){
if($id==null){
$user=$this->session::get('user');
if(isset($user['id']))
$id=$user['id'];
else
return false;
}
$res=$this->select("user","id=".$id);
return ($res && $res=$res->fetch_assoc())?$res:false;
}
function __destruct(){
if($this->result)
$this->result->close();
}
}
<?php
class config{
//Set database config setting
protected $host="localhost";
protected $db="gatetjaq_enotepad";
protected $user="gatetjaq_notepad";
protected $password="O_JcVSOI!Cg{";
public $base_url="https://9code.info/demo-oop/";
}
include(DIR."/Class/email.php");
<?php
class email{
function send($to,$sub,$message,$html=1,$params=null){
$headers = "From: [email protected]\r\n";
$headers .= "MIME-Version: 1.0\r\n";
if($html)
$headers .= "Content-Type: text/html; charset=UTF-8\r\n";
return mail($to,$sub,$message, $headers);
}
}
All class and files are created and hope you understand the each line code and not then comment me i will describe you via call or skype.
Now we creating login page/ login.php file
<?php
include("backend/init.php");
?>
<html lang="en"><head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1, shrink-to-fit=no">
<meta name="description" content="">
<meta name="author" content="">
<title>Login</title>
<?php
include("common/header.php");
?>
</head>
<body id="page-top">
<?php include("common/nav.php");?>
<!-- Masthead -->
<header class="masthead" style="
/* float: left; */
/* width: 100%; */
">
<div class="container ">
<div class="row align-items-center justify-content-center text-center">
<div class="col-lg-10 align-self-end">
<h1 class=" text-black font-weight-bold">Login</h1>
<hr class="divider my-4">
</div>
<div id="edit_content" class="col-lg-12 align-items-center">
<div class="note_form">
<form method="post" name="note_form" id="note_form" role="form" class="form-horizontal text-center" action="process.php" >
<div class="col-md-5 text-left" style="margin: auto;">
<?php $app->getMessages();?>
<div class="form-group">
<label for="uname" class="sr-only">User Name</label>
<input type="text" name="user_name" id="uname" class="form-control " placeholder="Email address" />
</div>
<div class="form-group">
<label for="password" class="sr-only">Password</label>
<input type="password" name="password" id="password" class="form-control " placeholder="Password" />
</div>
<div class="form-group">
<input type="hidden" name="action" value="login" />
<input type="submit" class="btn btn-lg btn-primary btn-block" value="Sign in" id="btnSaveNote" tabindex="3">
</div>
<div class="checkbox">
<label>
<a href="register.php" class="align-center">Register now if you new </a>
</label>
</div>
<div class="clear"><br/></div>
</div>
</form>
</div>
</div>
</div>
</div>
</header>
<?php
include("common/footer.php");
?>
</body></html>
Here we included two files
include(“common/header.php”);
include(“common/footer.php”);
include(“common/nav.php”);
In creating three files one by one
<!-- Font Awesome Icons -->
<link href="vendor/fontawesome-free/css/all.min.css" rel="stylesheet" type="text/css">
<!-- Google Fonts -->
<link href="https://fonts.googleapis.com/css?family=Merriweather+Sans:400,700" rel="stylesheet">
<link href="https://fonts.googleapis.com/css?family=Merriweather:400,300,300italic,400italic,700,700italic" rel="stylesheet" type="text/css">
<!-- Theme CSS - Includes Bootstrap -->
<link href="css/creative.css" rel="stylesheet">
<link rel="stylesheet" href="css/samples.css?a=a">
<link rel="stylesheet" href="toolbarconfigurator/lib/codemirror/neo.css">
<!-- Footer -->
<footer class="bg-light py-5">
<div class="container">
<div class=" text-center">
<div style="text-align: center">
<p class="stats">
Users - 000000001 | Recent Notes - 00000001
</p>
<p>© 2019 eNotepad</p>
</div>
<p>
<a href="/">Home</a>
| <a href="/privacy.php">Privacy</a>
| <a href="/about-us.php">About</a>
| <a href="/features.php">Features</a>
</p>
<p>
eNotepad is your online notepad on the web. It allows you to store notes on the GO without having to Login.
<br>
You can use a rich text editor, sort notes by date or title and make notes private.
<br>
Best of all - anotepad is a fast, clean, simple to use and FREE notepad online.
<br>
<br>
</p>
</div>
</div>
</footer>
<!-- Bootstrap core JavaScript -->
<script type="text/javascript" src="https://cdnjs.cloudflare.com/ajax/libs/jquery/1.11.0/jquery.min.js"></script>
<script src="vendor/bootstrap/js/bootstrap.bundle.min.js"></script>
<!-- Custom scripts for this template -->
<script src="js/creative.js"></script>
<?php
$user=$app->db->getUser();
?>
<!-- Navigation -->
<nav class="navbar navbar-expand-lg navbar-light py-3 bg-light" id="mainNav">
<div class="container">
<a class="navbar-brand " href="/">eNotepad</a>
<button class="navbar-toggler navbar-toggler-right" type="button" data-toggle="collapse" data-target="#navbarResponsive" aria-controls="navbarResponsive" aria-expanded="false" aria-label="Toggle navigation">
<span class="navbar-toggler-icon"></span>
</button>
<div class="collapse navbar-collapse" id="navbarResponsive" style="
/* background: #fff; */
">
<ul class="navbar-nav ml-auto my-2 my-lg-0">
<li class="nav-item">
<a class="nav-link " href="/">Home</a>
</li>
<li class="nav-item">
<a class="nav-link " href="features.php">Features</a>
</li>
<li class="nav-item">
<a class="nav-link " href="about-us.php">About US</a>
</li>
<li class="nav-item">
<?php if($user!=null):?>
<div class="dropdown show d-inline-block ">
<a class="btn btn-primary dropdown-toggle" href="#" role="button" id="dropdownMenuLink" data-toggle="dropdown" aria-haspopup="true" aria-expanded="false">
Welcome <?=ucfirst($user['name'])?></a>
<div class="dropdown-menu" aria-labelledby="dropdownMenuLink">
<a class="dropdown-item" href="process.php?action=logout">Logout</a>
</div>
</div>
<?php else:?>
<a class=" btn btn-primary" href="login.php">Login</a> | <a class=" btn btn-primary" href="register.php">Register</a>
<?php endif;?>
</li>
</ul>
</div>
</div>
</nav>
So login page is ready now
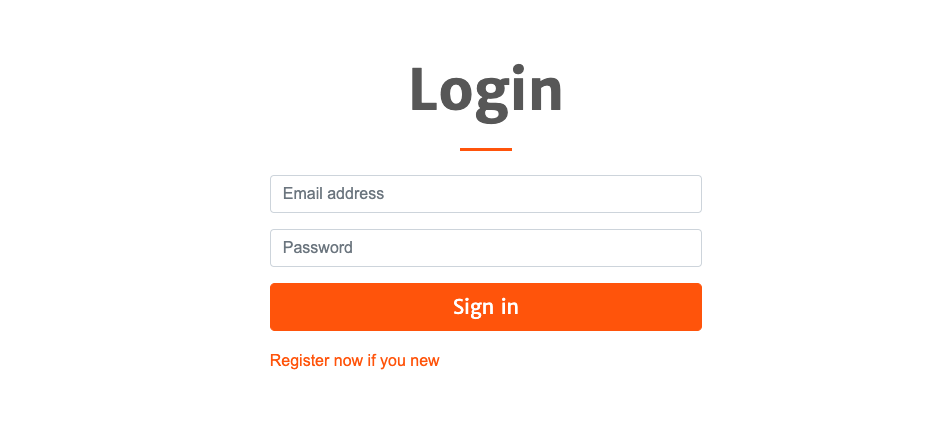
Here is login page output
Now we are going to add register.php page
<?php
include("backend/init.php");
?><html lang="en"><head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1, shrink-to-fit=no">
<meta name="description" content="">
<meta name="author" content="">
<title>eNotepad Demo</title>
<?php
include("common/header.php");
?>
</head>
<body id="page-top">
<?php include("common/nav.php");?>
<!-- Masthead -->
<header class="masthead" style="
/* float: left; */
/* width: 100%; */
">
<div class="container ">
<div class="row align-items-center justify-content-center text-center">
<div class="col-lg-10 align-self-end">
<h1 class=" text-black font-weight-bold">Register now</h1>
<p>Quick fill below information, It's safe and free</p>
<hr class="divider my-4">
</div>
<div id="edit_content" class="col-lg-12 align-self-end">
<div class="note_form">
<form method="post" name="note_form" id="note_form" role="form" class="form-horizontal" action="process.php" >
<div class="col-md-5 text-left" style="margin: auto;">
<?php $app->getMessages();?>
<div class="form-group">
<label for="name" class="sr-only">Full Name</label>
<input type="text" name="name" id="name" class="form-control " placeholder="Full Name"/>
</div>
<div class="form-group">
<label for="uname" class="sr-only">Email</label>
<input type="email" name="user_name" id="uname" class="form-control " placeholder="Email address" />
</div>
<div class="form-group">
<label for="password" class="sr-only">Password</label>
<input type="password" name="password" id="password" class="form-control " placeholder="Password" />
</div>
<div class="form-group">
<input type="hidden" name="action" value="register"/>
<input type="submit" class="btn btn-lg btn-primary btn-block" value="Register Now" id="btnSaveNote" tabindex="3">
</div>
<div class="checkbox">
<label>
<a href="login.php" class=""> Go to login page if you have already account.</a>
</label>
</div>
<div class="clear"><br/></div>
</div>
</form>
</div>
</div>
</div>
</div>
</header>
<?php
include("common/footer.php");
?></body></html>
Above code for generate register page
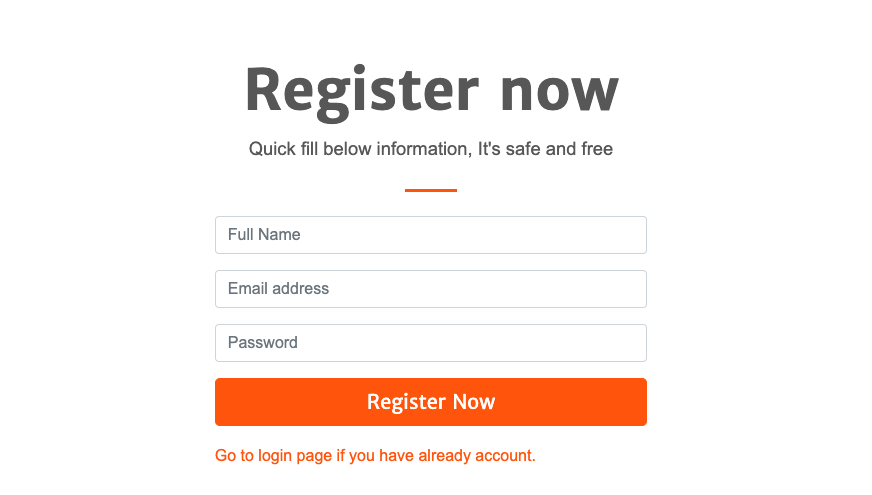
Now you can download or view demo by click below options
Thank you for visit and for more info please comment or drop me mail for more function if we can add.
Leave a Reply